WPF基础
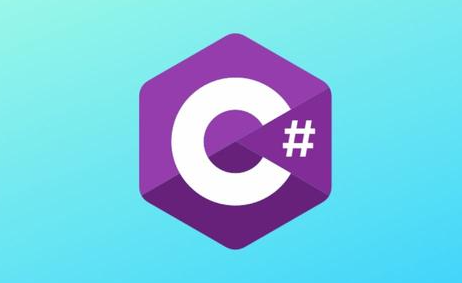
WPF基础
MR.XSSWPF
1、WPF栅格
WPF有着和BootStrap类似的栅格系统,可以将页面分割为你所需要的块大小,并且为响应式布局结构
示例,将页面分为3行3列,就如数组一样,下标从0开始,想使用某一个区域,直接指定Grid.Column ,Grid.Row 这两个属性即可,下面示例指向了中间区域。
1 | <Window x:Class="WPF基础学习.WPFTEST" |
2、Style
指定组件类型,类似CSS标签选择器,示例
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25<Window x:Class="WPF基础学习.WPFSTYLE"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPF基础学习"
mc:Ignorable="d"
Title="WPFSTYLE" Height="450" Width="800">
<Window.Resources>
<Style TargetType="Button">
<Setter Property="Background" Value="Green"/>
<Setter Property="FontSize" Value="20"/>
<Setter Property="Width" Value="100"/>
</Style>
</Window.Resources>
<Grid>
<StackPanel Orientation="Vertical">
<Label Content="style属性指向同一类型" Margin="330,0,0,0"/>
<Button Content="1"/>
<Button Content="2"/>
<Button Content="3"/>
</StackPanel>
</Grid>
</Window>设置单独的样式,类似于CSS的ID选择器
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34<Window x:Class="WPF基础学习.WPFIDSTYLE"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPF基础学习"
mc:Ignorable="d"
Title="WPFIDSTYLE" Height="450" Width="800">
<Window.Resources>
<Style x:Key="LOGINBUTTON" TargetType="Button">
<Setter Property="Background" Value="Gray"/>
<Setter Property="FontSize" Value="18"/>
<Setter Property="Width" Value="50"/>
</Style>
<Style x:Key="loginoutbutton" TargetType="Button">
<Setter Property="Background" Value="Green"/>
<Setter Property="FontSize" Value="18"/>
<Setter Property="Width" Value="50"/>
</Style>
</Window.Resources>
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="30"/>
<RowDefinition Height="30"/>
</Grid.RowDefinitions>
<Button Style="{StaticResource LOGINBUTTON}" Content="登录" Grid.Row="0" Grid.Column="0"/>
<Button Style="{StaticResource loginoutbutton}" Content="登录" Grid.Row="1" Grid.Column="0"/>
</Grid>
</Window>单独设置样式文件,引入
新建样式资源文件
编写外部样式
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27<ResourceDictionary xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml">
<Style TargetType="Button">
<Setter Property="Background" Value="White"/>
<Setter Property="FontSize" Value="18"/>
<Setter Property="Width" Value="50"/>
</Style>
<Style x:Key="LOGINBUTTON" TargetType="Button">
<Setter Property="Background" Value="Gray"/>
<Setter Property="FontSize" Value="18"/>
<Setter Property="Width" Value="50"/>
</Style>
<Style x:Key="loginoutbutton" TargetType="Button">
<Setter Property="Background" Value="Green"/>
<Setter Property="FontSize" Value="18"/>
<Setter Property="Width" Value="50"/>
</Style>
<Style x:Key="extends" TargetType="Button" BasedOn="{StaticResource {x:Type Button}}">
<Setter Property="Height" Value="60"/>
<Setter Property="Width" Value="60"/>
<Setter Property="Background" Value="Blue"/>
</Style>
</ResourceDictionary>全局引入
1
2
3
4
5
6
7
8
9
10
11
12
13<Application x:Class="WPF基础学习.App"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WPF基础学习"
StartupUri="WPFIDSTYLE.xaml">
<Application.Resources>
<ResourceDictionary>
<ResourceDictionary.MergedDictionaries>
<ResourceDictionary Source="basebutton.xaml"/>
</ResourceDictionary.MergedDictionaries>
</ResourceDictionary>
</Application.Resources>
</Application>
3、数据绑定
在使用WPF时,数据交互遵从的和Vue类似,使用数据绑定,来实现页面交互,下面代码示例
页面代码
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37<Window x:Class="WPF基础学习.Login1"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:WPF基础学习"
mc:Ignorable="d"
Title="登录" Height="450" Width="800">
<Grid>
<Grid.RowDefinitions>
<RowDefinition Height="35"/>
<RowDefinition/>
</Grid.RowDefinitions>
<StackPanel Grid.Row="0" Background="#0c7ece">
<TextBlock Grid.Row="0" Text="登录" HorizontalAlignment="Center" FontSize="20" Margin="5"/>
</StackPanel>
<Grid Grid.Row="1">
<Grid.RowDefinitions>
<RowDefinition Height="30"/>
<RowDefinition Height="30"/>
<RowDefinition Height="30"/>
</Grid.RowDefinitions>
<StackPanel Orientation="Horizontal" Grid.Row="0" HorizontalAlignment="Center">
<TextBlock Text="用户名" Margin="5" />
<TextBox Text="{Binding Username}" Width="100" Height="20"/>
</StackPanel>
<StackPanel Orientation="Horizontal" Grid.Row="1" HorizontalAlignment="Center">
<TextBlock Text="密码" Margin="5" />
<TextBox Text="{Binding Password}" Width="100" Height="20"/>
</StackPanel>
<Button Grid.Row="2" x:Name="Login" Content="登录" Click="Login_" Height="30" Width="100"/>
</Grid>
</Grid>
</Window>页面图
后端代码
注意,在前端使用binding与属性绑定之后,数据只是单向的(前端 –> 后端),如果实现INotifyPropertyChanged接口,重写方法,并在setter里面调用该方法,与属性值进行绑定,此时,数据 后端 –> 前端 这个通道才是通的
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Shapes;
namespace WPF基础学习
{
/// <summary>
/// Login1.xaml 的交互逻辑
/// </summary>
public partial class Login1 : Window, INotifyPropertyChanged
{
public Login1()
{
InitializeComponent();
// 数据绑定
this.DataContext = this;
}
//数据双向绑定
public event PropertyChangedEventHandler PropertyChanged;
private void RaisePropertyChaged(string propertyName)
{
PropertyChangedEventHandler handler = PropertyChanged;
if (handler != null)
{
handler(this, new PropertyChangedEventArgs(propertyName));
}
}
private string _Username;
public string Username
{
get { return _Username; }
set
{
_Username = value;
//调用该方法,将数据 后端 --> 前端 形成通路
RaisePropertyChaged("Username");
}
}
private String _Password;
public String Password
{
get { return _Password; }
set
{
_Password = value;
RaisePropertyChaged("Password");
}
}
private void Login_(object sender, RoutedEventArgs e)
{
if (Username == null || Password == null)
{
MessageBox.Show("请输入用户名或者密码!");
}
else if (Password.Equals("123") && Username.Equals("admin"))
{
Test test = new Test();
test.Show();
this.Close();
}
else
{
MessageBox.Show("用户名或者密码错误!");
Username = "";
Password = "";
}
}
}
}
4、MVVM
MVVM(Model-View-ViewModel)是一种设计模式,特别适用于WPF(Windows Presentation Foundation)等XAML-based的应用程序开发。MVVM模式主要包含三个部分:Model(模型)、View(视图)和ViewModel(视图模型)。
- Model(模型):模型代表的是业务逻辑和数据。它包含了应用程序中用于处理的核心数据对象。模型通常包含业务规则、数据访问和存储逻辑。
- View(视图):视图是用户看到和与之交互的界面。在WPF中,视图通常由XAML定义,并且包含各种用户界面元素,如按钮、文本框、列表等。
- ViewModel(视图模型):视图模型是视图的抽象,它包含视图所需的所有数据和命令。视图模型通过实现
INotifyPropertyChanged
接口和使用ICommand
对象,将视图的状态和行为抽象化,从而实现了视图和模型的解耦。
MVVM模式的主要优点是分离了视图和模型,使得视图和业务逻辑之间的依赖性降低,提高了代码的可维护性和可测试性。此外,通过数据绑定和命令绑定,MVVM模式可以减少大量的样板代码,使得代码更加简洁和易于理解。
Comment
匿名评论隐私政策